[v.1.0] (09/15/2023): Post started!
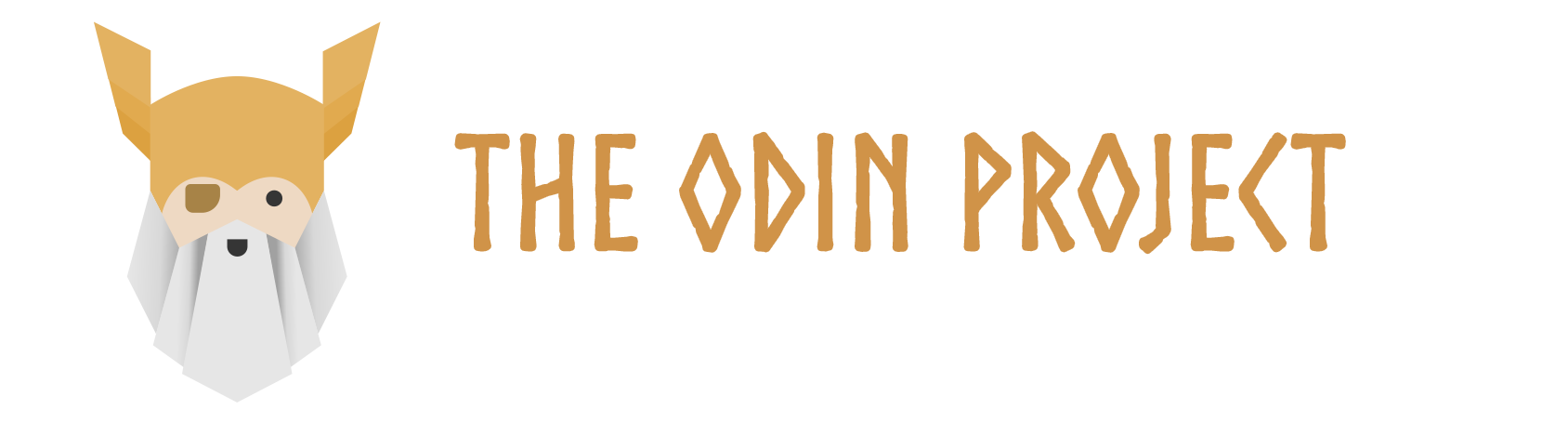
Fundamentals
- The Rubber-Duck method:
- What do you think the problem is?
- What exactly do you want to happen?
- What is actually happening?
- How did you get there?
- What have you tried so far?
Web Development
- Why Learning to Code is So Damn Hard
- Quora: How can I Become a Really Good Web Developer?
- Quora: What makes a great web developer?
- Jared the Nerd: What makes a good Developer?
- FreeCodeCamp: Things I Wish Someone Had Told Me When I Was Learning How To Code
- TechCrunch: Don’t Believe Anyone who Tells you Learning to Code is Easy
- Code Quizzes: Deliberate Programming Practice
Mindsets
- Believe you can get better
- Grit
- You can learn anything
- How to Learn Podcast
- Becoming a TOP Success Story
- Managing inspiration and motivation
- Learning to code when it gets dark
- Why Procrastinators Procrastinate Learn about the Instant Gratification Monkey, Rational Decision Maker, Panic Monster, and how to navigate the Dark Playground
Asking for Help
- Don’t ask to ask, just ask
- The XY Problem
- How to ask technical questions
- How to use Google to solve your programming questions
- How to be great at asking coding questions
Computer Fundamentals
- Watch this BBC short for an overview of how the internet works.
- Read this article from Mozilla on “How does the Internet work?”.
- Watch How the Internet Works in 5 Minutes.
- Read up on the differences between a web page, a web server, and a search engine.
- Watch this Google short explaining what a web browser is. Then, find out what web browser you are using right now.
- Read about how one part of the web interacts with another and read about or watch a DNS request in action.
Command Lines
- The Art of Command Line is a complete beginner’s pro-maker. It serves as an open-source repository. This also has a lot of pro tips!
- ExplainShell.com is a great resource if you want to deconstruct a particularly strange shell command or learn how Bash works through guess-and-check.
- Command Line Flashcards by flashcards.github.io.
- Linux Commands for Beginners contains 24 videos explaining the basics of the command line. Videos are brief enough for beginners but, at the same time, detailed enough to get you started and light your inner curiosity.
Git
- Understanding SSH Key Pairs SSH is a secure network protocol that uses an implementation of public-key cryptography, also known as asymmetric cryptography. Having a basic understanding of how it works can help you understand what an SSH key is all about.
- Asymmetric Encryption - Simply explained a short video explaining Asymmetric Encryption.
- Git - Reference
- How to Write a Git Commit Message
- Conventional commits
- Conventional commits
HTML, CSS, & Flexbox
- To validate html code, use validator.
HTML
- Bookmark DevDocs.io Read the “Welcome” message. Massive API documentation collection that even works offline. An essential collection of reference material for everything covered and more.
- Don’t Fear the Internet’s video about HTML
- When to Use
<strong>
,<b>
,<em>
, and<i>
Tags in Your Markup - MDN documentation on the unordered list element
- MDN documentation on the ordered list element
- Shay Howe’s HTML lists tutorial
- Interneting is hard’s treatment on HTML links and images
- What happened the day Google decided links including (/) were malware
- Chris Coyier’s When to use target=”_blank” on CSS-Tricks
CSS
-
Specificity will only be taken into account when an element has multiple, conflicting declarations targeting it, sort of like a tie-breaker. An ID selector will always beat any number of class selectors, a class selector will always beat any number of type selectors, and a type selector will always beat any number of less specific selectors. When no declaration has a selector with a higher specificity, a larger amount of a single selector will beat a smaller amount of that same selector.
-
Mozilla CSS values and units can be used to learn the various types of values possible in absolute or relative terms.
-
An interactive Scrim which covers much of the material in the lesson in an interactive form.
-
The CSS Cascade is a great, interactive read that goes a little more in detail about other factors that affect what CSS rules actually end up being applied.
-
Changing the Font Family describes a few different approaches to using custom fonts.
-
CSS Specificity Explained from Kevin Powell goes through various specificity examples and gives some advice on avoiding wrestling with specificity.
-
CSS Specificity Calculator allows you to fill in your own selectors and have their specificity calculated and visualized.
-
Mozilla CSS Properties Reference can be used to learn if a particular CSS property is inherited or not; simply look for the Inherited field inside the Formal Definition section. Here’s an example for the CSS color property.
The Box Model
-
The only real complication here is that there are many ways to manipulate the size of these boxes, and the space between them, using padding, margin, and border. The assigned articles go into more depth on this concept, but to sum it up briefly:
padding
increases the space between the border of a box and the content of the box.margin
increases the space between the borders of a box and the borders of adjacent boxes.border
adds space (even if it’s only a pixel or two) between the margin and the padding.
-
This CSS Tricks page has some further information about the margin property that you’ll find useful. Specifically, the sections about auto and margin collapsing contain things you’ll want to know.
-
For a more interactive explanation and example, try this Scrim on the box model.
-
Watch “this” simple short video on What does the term “Normal Flow” Mean In CSS
-
For a more interactive explanation and example, try this Scrim on block and inline display.
Flexbox
-
Flexbox gave us a ton of amazing new tools for laying out a web page. Compare these techniques to what we were able to do with floats, and it should be pretty clear that flexbox is a cleaner option for laying out modern websites:
- Use
display: flex;
to create a flex container. - Use
justify-content
to define the horizontal alignment of items. - Use
align-items
to define the vertical alignment of items. - Use
flex-direction
if you need columns instead of rows. - Use the
row-reverse
orcolumn-reverse
values to flip item order. - Use
order
to customize the order of individual elements. - Use
align-self
to vertically align individual items. - Use
flex
to create flexible boxes that can stretch and shrink.
- Use
-
This Flexbox tutorial is a friendly tutorial for modern CSS layouts by Interneting Is Hard.
-
For a more interactive explanation and example, try this Scrim on Flexbox. Note that this Scrim requires logging into Scrimba in order to view.
-
Check out this video explaining how flexbox works and why.
-
For an interactive explanation and demo, check out the Scrim on the flex shorthand. For an alternative explanation you can view the Scrim on using flex-grow, flex-shrink, and flex-basis. Note that these Scrims require logging into Scrimba in order to view.
-
This flexbox visual cheatsheet has some useful references to flex and its properties.
-
For an interactive demo, check out this Scrim on Flexbox axes. Note that this Scrim requires logging into Scrimba in order to view.
-
This beautiful Interactive Guide to Flexbox covers everything you need to know. It will help reinforce concepts we’ve already touched on with some really fun and creative examples. Spend some time here, some of it should be review at this point, but the foundations here are important!
-
Typical use cases of Flexbox is an MDN article that covers some more practical tips. Don’t skip the interactive sections! Playing around with this stuff is how you learn it!
-
The CSS Tricks “Guide to Flexbox” is a classic. There isn’t any new information for you here, but the images and examples are super helpful. This one is a great cheat sheet that you’ll probably return to often. (Keep it handy for the practice exercises!)
-
Flexbox Froggy is a funny little game for practicing moving things around with flexbox.
-
Flexbox Zombies is another gamified take on flexbox. Free, but requires an account.
-
The Basic Concepts of Flexbox article on MDN is another good starting point. There are helpful examples and interactive sections.
-
Aligning Items in a Flex Container goes into more depth on the topic of axes and
align-items
vsjustify-content
. -
This Flexbox Tutorial from freecodecamp is another decent resource.
-
Flexbox Crash Course is a nice resource by Traversy Media.
-
For more interactive demos, try the Scrim on the
justify-content
property and the Scrim on thealign-items
property. Note that these Scrims require logging into Scrimba in order to view.
JavaScript
- The differences between var and let are explained in this javascript.info article titled the old “var”.
- Data types in JavaScript.
- Handling text — strings in JavaScript
- Regular expressions, commonly known as regex, is a tool that matches or locates patterns in strings for string validation. However, it’s important to know when you shouldn’t use regular expressions. There are other various methods to process strings, and regex can be slower in comparison.
- Web Dev Simplified’s Regular Expressions In 20 Minutes
- Chrome DevTools Documentation by Google.
- View and change CSS
- CSS features reference
- Get Started With Viewing And Changing The DOM
- Simulate mobile devices with Device Mode
- Debug JavaScript
- Pause your code with breakpoints
- The console overview video and read through the page to familiarize yourself with the console and its usage.
- Learn 14 tips and tricks in this JavaScript 30 Video by Wes Bos
- Functions
- Function expressions
- Arrow functions, the basics
- JavaScript Call Stack
- What’s the difference between using “let” and “var”? - stackoverflow
- How JavaScript Code is executed?
- The Art of Problem Solving
- Read How to Think Like a Programmer - Lessons in Problem Solving by Richard Reis.
- Watch How to Begin Thinking Like a Programmer by Coding Tech. It’s an hour long but packed full of information and definitely worth your time watching.
- Read this Pseudocode: What It Is and How to Write It article from Built In.
- Read the first chapter in Think Like a Programmer: An Introduction to Creative Problem Solving (not free). This book’s examples are in C++, but you will understand everything since the main idea of the book is to teach programmers to better solve problems. It’s an amazing book and worth every penny. It will make you a better programmer.
- Watch this video on repetitive programming techniques.
- Watch Jonathan Blow on solving hard problems where he gives sage advice on how to approach problem solving in software projects.
- MDN Javascript Errors Reference
- This list of clean-code tips.
- This article, and this one too about the role of comments in your code.
- A nice op-ed
- Airbnb style guide
- Chaining methods to write sentences
- This article covers some of the most useful built-in array methods. These fundamentals are something you’ll use every day, so don’t rush too much and miss out!
- The Document Object Model (DOM) is an application programming interface (API) for manipulating HTML documents. The DOM represents an HTML document as a tree of nodes. The DOM provides functions that allow you to add, remove, and modify parts of the document effectively.
- Eloquent JS - DOM
- Eloquent JS - Handling Events
- DOM Enlightenment
- Plain JavaScript is a reference of JavaScript code snippets and explanations involving the DOM, as well as other aspects of JS. If you’ve already learned jQuery, it will help you figure out how to do things without it.
- This W3Schools article offers simple and easy-to-understand lessons on the DOM.
- JS DOM Crash Course is an extensive and well explained 4 part video series on the DOM by Traversy Media.
- Understanding The Dom is an aptly named article-based tutorial series by DigitalOcean.
- Introduction to events by MDN covers the same topics you learned in this lesson on events.
- Wes Bos’s Drumkit JavaScript30 program reinforces the content covered in the assignment. In the video you will notice that a deprecated keycode keyboard event is used, replace it with the recommended code keyboard event and replace the data-key tags accordingly.
- Event Capture, Propagation and Bubbling video from Wes Bos’s JavaScript30 program.
- Actively learn the Git workflows discussed in this lesson with this interactive Visual Git Cheatsheet by Andrew Peterson. It’s okay to be unfamiliar with the variety of commands you’ll interact with. You’ll learn about them later in the curriculum.
- Make pushing your local commits to remote branches easier with the command git push -u origin
. It automatically links the local branch you push with the remote one. Read this educative.io article by Talha Ashar and commit faster to a remote branch with a simple git push command. - Learn Git Branching by going through this interactive visualizer by Peter Cottle. You can learn the substantial commands about branching while seeing how the commands you write affect your visually presented branch tree.
Citation
Cited as:
Odin's Resources Repository https://mnguyen0226.github.io/posts/web_dev_odin/post/.\
Or
@article{nguyen2023odin,
title = "Odin's Resources Repository",
author = "Nguyen, Minh",
journal = "mnguyen0226.github.io",
year = "2023",
month = "September",
url = "https://mnguyen0226.github.io/posts/web_dev_odin/post/"
}
References
[1] Full Stack JavaScript | The Odin Project. Theodinproject.com. Published 2023. Accessed September 15, 2023. https://www.theodinproject.com/paths/full-stack-javascript
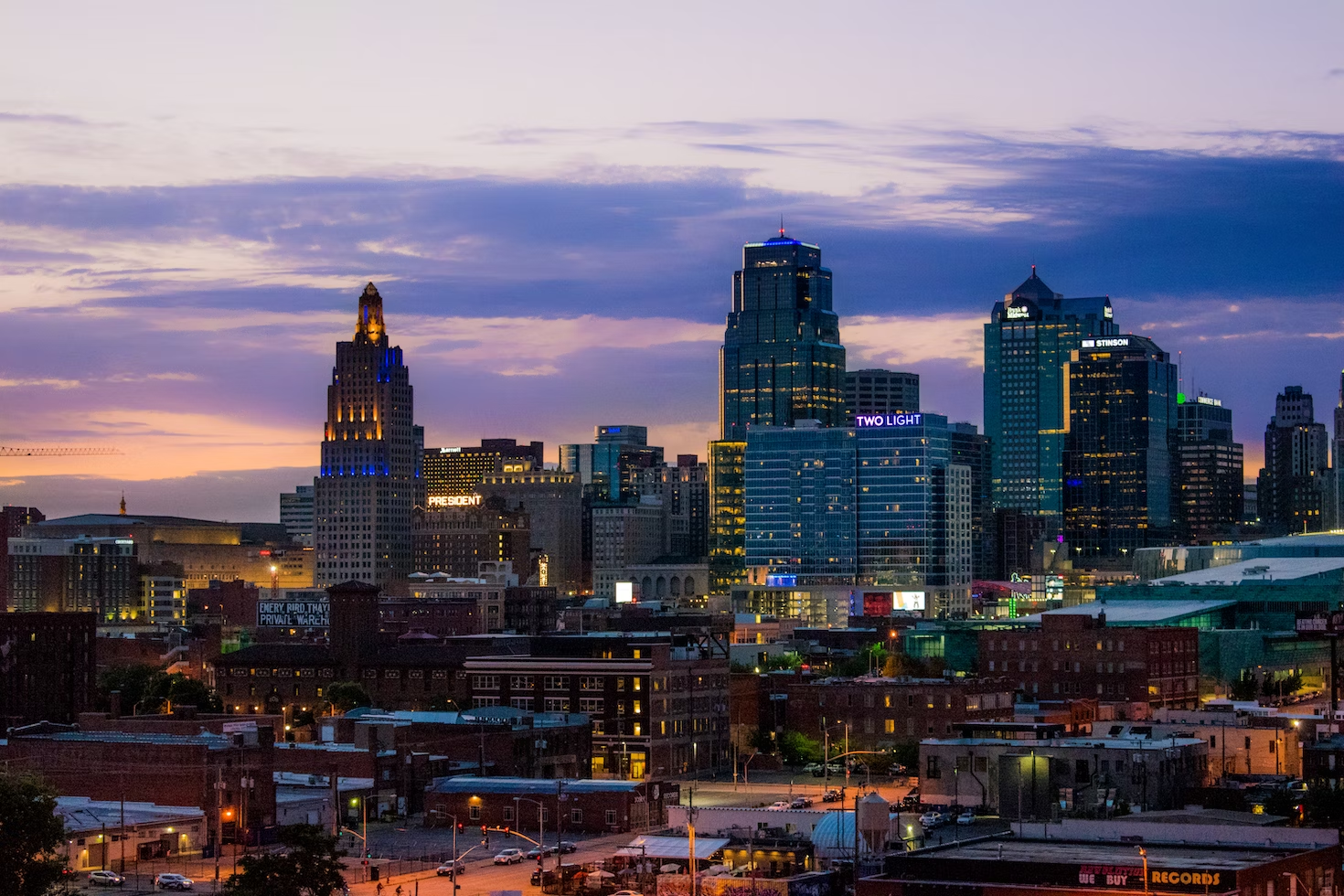